Java Platform
The Java platform is a suite of programs that facilitate developing and running programs written in the Java programming language. The platform is not specific to any one processor or operating system, rather an execution engine (called a virtual machine) and a compiler with a set of libraries are implemented for various hardware and operating systems so that Java programs can run identically on all of them. There are multiple platforms, each targeting a different class of devices:
Java Card : A technology that allows small Java-based applications (applets) to be run securely on smart cards and similar small-memory devices.
Java ME (Micro Edition): Specifies several different sets of libraries (known as profiles) for devices with limited storage, display, and power capacities. Often used to develop applications for mobile devices, PDAs, TV set-top boxes, and printers.
Java SE(Standard Edition) : For general-purpose use on desktop PCs, servers and similar devices.
Java EE (Enterprise Edition) : Java SE plus various APIs useful for multi-tier client–server enterprise applications.
The Java platform consists of several programs, each of which provides a portion of its overall capabilities. For example, the Java compiler, which converts Java source code into Java bytecode (an intermediate language for the JVM), is provided as part of the Java Development Kit (JDK). The Java Runtime Environment (JRE), complementing the JVM with a just-in-time (JIT) compiler, converts intermediate bytecode into native machine code on the fly. An extensive set of libraries are also part of the Java platform.
The essential components in the platform are the Java language compiler, the libraries, and the runtime environment in which Java intermediate bytecode executes according to the rules laid out in the virtual machine specification.
Java Virtual Machine
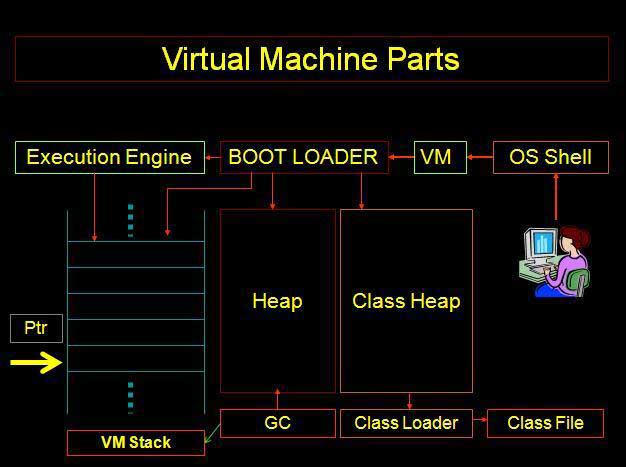
The heart of the Java platform is the concept of a "virtual machine" that executes Java bytecode programs. This bytecode is the same no matter what hardware or operating system the program is running under. There is a JIT (Just In Time) compiler within the Java Virtual Machine, or JVM. The JIT compiler translates the Java bytecode into native processor instructions at run-time and caches the native code in memory during execution.
The use of bytecode as an intermediate language permits Java programs to run on any platform that has a virtual machine available. The use of a JIT compiler means that Java applications, after a short delay during loading and once they have "warmed up" by being all or mostly JIT-compiled, tend to run about as fast as native programs.[citation needed] Since JRE version 1.2, Sun's JVM implementation has included a just-in-time compiler instead of an interpreter.
Class libraries
The Java class libraries serve three purposes within the Java platform. First, like other standard code libraries, the Java libraries provide the programmer a well-known set of functions to perform common tasks, such as maintaining lists of items or performing complex string parsing. Second, the class libraries provide an abstract interface to tasks that would normally depend heavily on the hardware and operating system. Tasks such as network access and file access are often heavily intertwined with the distinctive implementations of each platform. The java.net and java.io libraries implement an abstraction layer in native OS code, then provide a standard interface for the Java applications to perform those tasks. Finally, when some underlying platform does not support all of the features a Java application expects, the class libraries work to gracefully handle the absent components, either by emulation to provide a substitute, or at least by providing a consistent way to check for the presence of a specific feature.
Free software
Sun announced in JavaOne 2006 that Java would become free and open source software, and on October 25, 2006, at the Oracle OpenWorld conference, Jonathan I. Schwartz said that the company was set to announce the release of the core Java Platform as free and open source software within 30 to 60 days
Sun released the source code of the Class library under GPL on May 8, 2007, except some limited parts that were licensed by Sun from 3rd parties who did not want their code to be released under a free software and open-source license. Some of the encumbered parts turned out to be fairly key parts of the platform such as font rendering and 2D rasterising, but these were released as open-source later by Sun (see OpenJDK Class library).
Sun's goal was to replace the parts that remain proprietary and closed-source with alternative implementations and make the class library completely free and open source. In the meantime, a third party project called IcedTea created a completely free and highly usable JDK by replacing encumbered code with either stubs or code from GNU Classpath. Although OpenJDK has since become buildable without the encumbered parts (from OpenJDK 6 b10), IcedTea is still used by the majority of distributions, such as Fedora, RHEL, Debian, Ubuntu, Gentoo, Arch Linux and Slackware, as it provides security releases and an easier means for patch inclusion. OpenJDK also still doesn't include a browser plugin & Web Start implementation, so IcedTea's companion project, IcedTea-Web, is needed to fill this gap.
Compile/Execute Java Programs
Create a temporary folder C:\mywork. Using Notepad or another text editor, create a small Java file HelloWorld.java with the following text:
public class HelloWorld
{
public static void main(String[] args)
{
System.out.println("Hello, World!");
}
}
Save your file as HelloWorld.java in C:\mywork. To make sure your file name is HeloWorld.java, (not HelloWorld.java.txt), first choose "Save as file type:" All files, then type in the file name HelloWorld.java.
Run Command Prompt (found under All Programs/Accessories in the Start menu). Type
C:\> cd \mywork
This makes C:\mywork the current directory.
C:\mywork> dir
This displays the directory contents. You should see HelloWorld.java among the files.
C:\mywork> set path=%path%;C:\Program Files\Java\jdk1.5.0_09\bin
This tells the system where to find JDK programs.
C:\mywork> javac HelloWorld.java
This runs javac.exe, the compiler. You should see nothing but the next system prompt...
C:\mywork> dir
javac has created the HelloWorld.class file. You should see HelloWorld.java and HelloWorld.class among the files.
C:\mywork> java HelloWorld
This runs the Java interpreter. You should see the program output:
Hello, World!
If the system cannot find javac, check the set path command. If javac runs but you get errors, check your Java text. If the program compiles but you get an exception, check the spelling and capitalization in the file name and the class name and the java HelloWorld command. Java is case-sensitive!
It is possible to make the path setting permanent but you have to be very careful because your system might crash if you make a mistake. Proceed with extreme caution!
In Windows XP, go to Control Panel, choose "System," click on the "Advanced" tab, click on the "Environment variables" button. In the lower list, "System variables," click on Path:
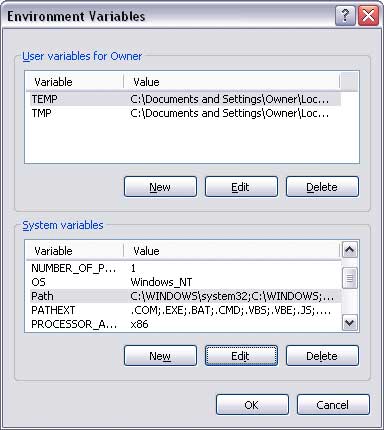
Click "Edit" and at the end append
;C:\Program Files\Java\jdk1.5.0_09\bin
(or the path to the appropriate folder where the latest version of JDK is installed). Do not put spaces before the appended path string.
Click OK on the path edit box and OK on the Ennvironment Variables box. The new setting will go into effect next time you run Command Prompt.
Java Basic Syntax
public class MyFirstJavaProgram {
/* This is my first java program.
* This will print 'Hello World' as the output
*/
public static void main(String []args) {
System.out.println("Hello World"); // prints Hello World
}
}
Open notepad and add the code as above.
Save the file as: MyFirstJavaProgram.java.
Open a command prompt window and go o the directory where you saved the class. Assume it's C:\.
Type ' javac MyFirstJavaProgram.java ' and press enter to compile your code. If there are no errors in your code, the command prompt will take you to the next line (Assumption : The path variable is set).
Now, type ' java MyFirstJavaProgram ' to run your program.
You will be able to see ' Hello World ' printed on the window.
C : > javac MyFirstJavaProgram.java
C : > java MyFirstJavaProgram
Hello World
Difference between JDK, JRE and JVM
JVM
JVM (Java Virtual Machine) is an abstract machine. It is a specification that provides runtime environment in which java bytecode can be executed. JVMs are available for many hardware and software platforms. JVM, JRE and JDK are platform dependent because configuration of each OS differs. But, Java is platform independent.
The JVM performs following main tasks:
1. Loads code
2. Verifies code
3. Executes code
4. Provides runtime environment
JRE
JRE is an acronym for Java Runtime Environment.It is used to provide runtime environment.It is the implementation of JVM.It physically exists.It contains set of libraries + other files that JVM uses at runtime.
Implementation of JVMs are also actively released by other companies besides Sun Micro Systems.
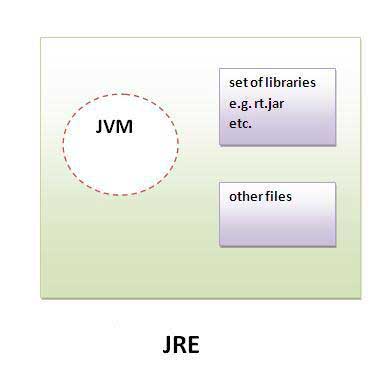
JDK
JDK is an acronym for Java Development Kit.It physically exists.It contains JRE + development tools.
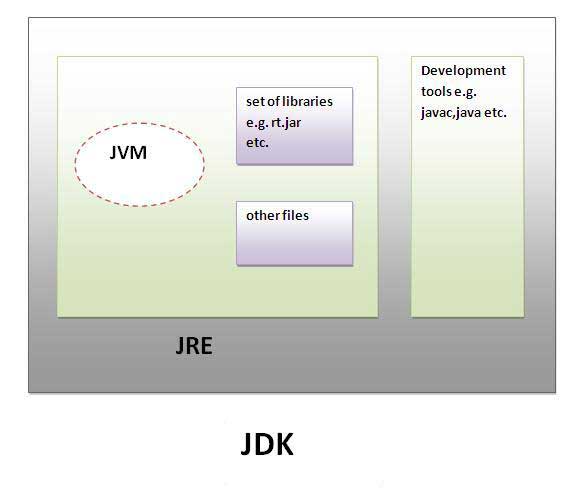
Variable and Datatype
Variable is a name of memory location. There are three types of variables: local, instance and static. There are two types of datatypes in java, primitive and non-primitive.
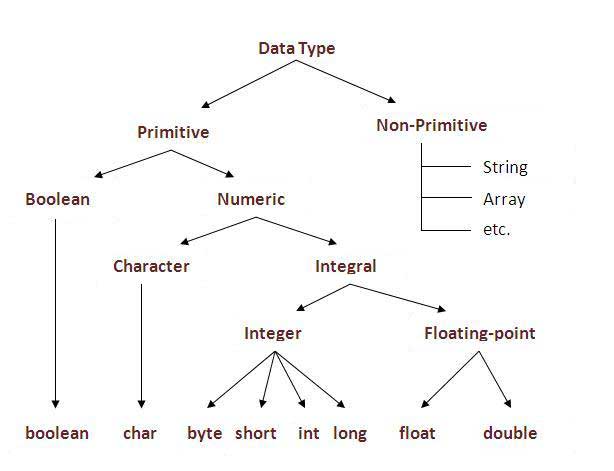
Java OOPs Concepts
Object Oriented Programming is a paradigm that provides many concepts such as inheritance, data binding, polymorphism etc.
OOPs (Object Oriented Programming System)
Object means a real word entity such as pen, chair, table etc. Object-Oriented Programming is a methodology or paradigm to design a program using classes and objects. It simplifies the software development and maintenance by providing some concepts:
1. Object
2. Class
3. Inheritance
4. Polymorphism
5. Abstraction
6. Encapsulation
Object : Any entity that has state and behavior is known as an object. For example: chair, pen, table, keyboard, bike etc. It can be physical and logical.
Class : Collection of objects is called class. It is a logical entity.
Inheritance : When one object acquires all the properties and behaviours of parent object i.e. known as inheritance. It provides code reusability. It is used to achieve runtime polymorphism.
Polymorphism : When one task is performed by different ways i.e. known as polymorphism. For example: to convense the customer differently, to draw something e.g. shape or rectangle etc.
Abstraction : Hiding internal details and showing functionality is known as abstraction. For example: phone call, we don't know the internal processing.
Encapsulation : Binding (or wrapping) code and data together into a single unit is known as encapsulation. For example: capsule, it is wrapped with different medicines.